Getting Started
Get Your Auth Token
Before you can get started with identity acceptance in your application, you must obtain and securely store your authentication token. Auth tokens are scoped to "Apps". Follow these steps to find your auth token:
- Visit dashboard.trinsic.id.
- Sign in to your account using your business email. (If you're not already onboarded, you can signup yourself or schedule an onboarding call here.)
- In the top navigation bar, you'll see your Organization's list of Apps. Select the App whose auth token you want to find.
- Go to the Settings page, and locate your auth token.
- Click on the auth token preview to copy it to your clipboard.
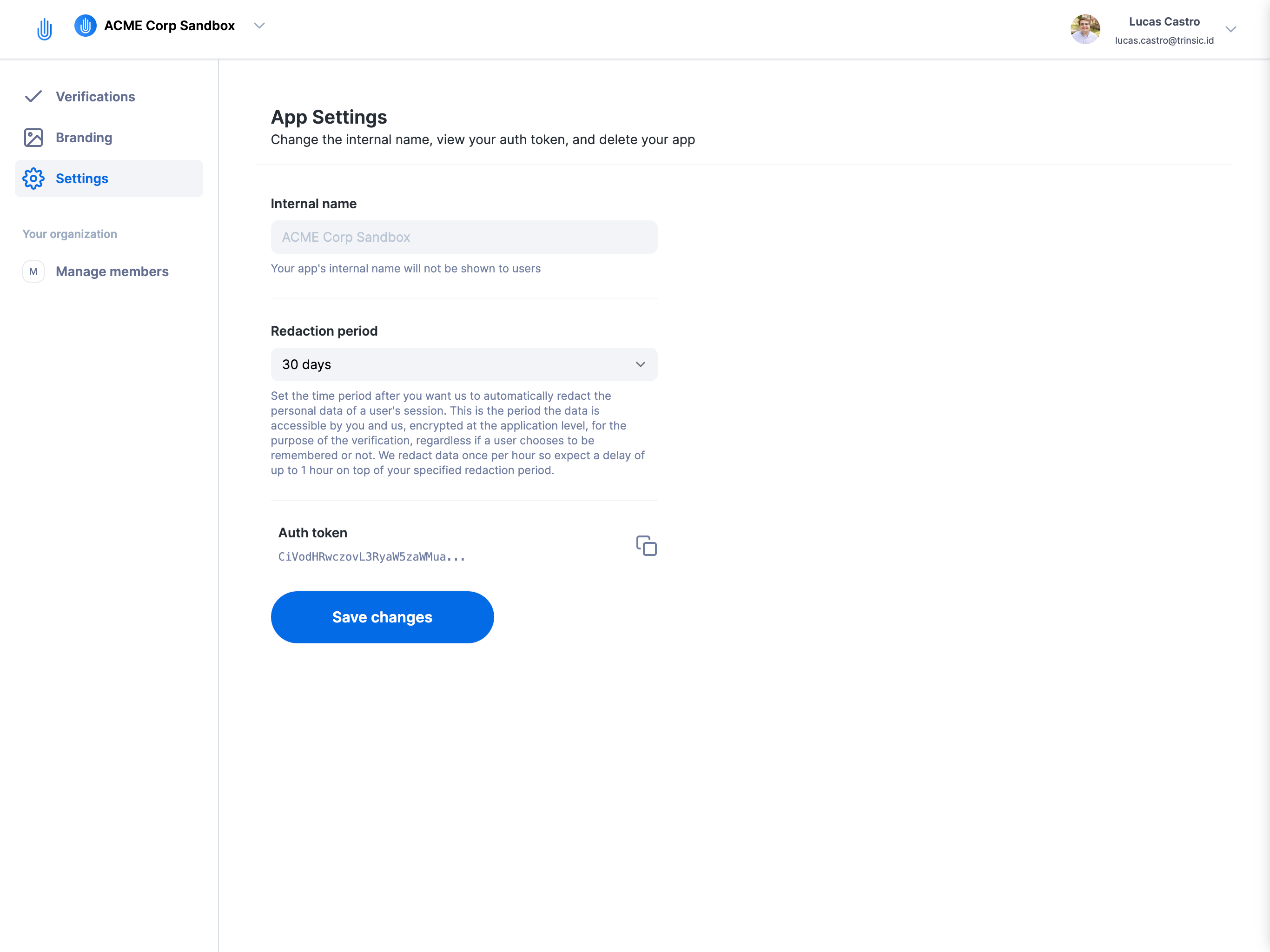
The auth token is everything you need to start integrating Trinsic in your application. But before you integrate, you'll probably want to customize Trinsic with your brand.
Other Application Settings
Besides retrieving your application's authentication token, you can also set the following options in the Settings page:
- Internal name: Your application's internal name. This name is used by you to quickly identify different applications in your organization. This name will never be shown to your users.
- Redaction period: How long you are able to view the sensitive identity output of a session. After a session gets redacted, you will still be able to see its final state; however, any PII will be deleted.
Customize Your Brand
If you are using the Trinsic Widget, you will want to customize it to create a more seamless user experience within your application. To customize the widget go to the Branding page on dashboard.trinsic.id
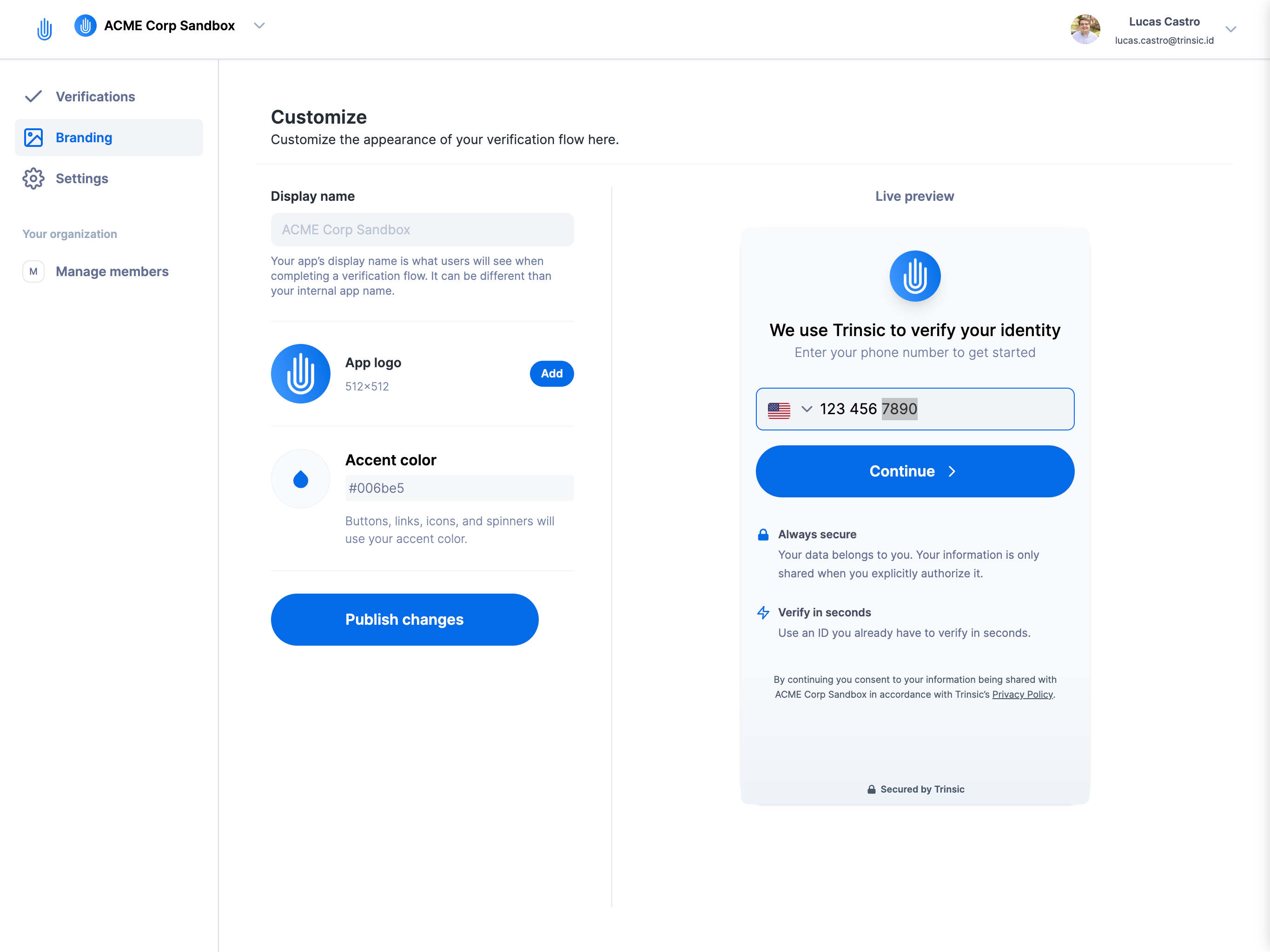
Branding options include:
- Display name: The recognizable name of your organization that will be shown to your users during verification.
- Logo: An image file of your company’s logo (recommended size of at least 512x512px).
- Accent color: The color associated with your brand to be used during the verification flow.
As you change these values, you will see live updates on the right and get a preview of how the widget will be displayed.
After customizing your brand, click the “Save changes”. Congratulations! You have successfully tailored your brand for a distinct and engaging user experience. Now, you are ready to integrate with the SDK.
Integrate with the SDK
Building a verification flow using Trinsic involves two parts: the server (API) and the client (UI). We support many backend and frontend stacks, but for now, let's look at how to implement Trinsic using Node.js with Express.
-
On your server side, instantiate the Trinsic service through the SDK by providing your account’s authentication token.
// On your backend import { Configuration, SessionsApi } from "@trinsic/api"; const config = new Configuration({ accessToken: "<your-auth-token>" }); const sessions = new SessionsApi(config);
-
On your backend, set up an endpoint to create a new session. This endpoint creates a new Acceptance Session with the Trinsic SDK whenever called and returns its launch URL to the client.
// On your backend const app = express(); app.post("/create-session", async (req, res) => { const result = await sessions.createWidgetSession(); return res.json({launchUrl: result.launchUrl}); });
-
On your backend, set up an endpoint to retrieve an acceptance session using the SDK. The client will need to supply the ID of the specific session, as well as the results access key that the client library returns at the end of a successful verification.
// On your backend app.post("/exchange-result", async (req, res) => { const { sessionId, accessKey } = req.body; const result = await sessionsApi.getSessionResult(sessionId, { resultsAccessKey: accessKey, }); return res.json(result); });
-
On your frontend, add the following package to your app:
npm i @trinsic/web-ui
-
On your frontend, add the following event handler and attach it to a trigger element:
// On your frontend import { launchIframe } from "@trinsic/web-ui"? const verifyButton = document.getElementById("verifyButton"); verifyButton.addEventListener("click", async () => { const { launchUrl } = await fetch("/create-session", { method: "POST" }).then((response) => response.json()); const result = await launchIframe(launchUrl); const data = await fetch("/exchange-result", { method: "POST", headers: { "Content-Type": "application/json" }, body: JSON.stringify({ sessionId: result.sessionId, accessKey: result.resultsAccessKey } }).then((response) => response.json()); console.log(data); });
Visualize Your Verifications
Finally, let's explore the Verifications page on your dashboard. This page displays all verification sessions you have created, alongside their status and creation/update timestamps. Successful verifications which have not yet been redacted can be inspected to view the resultant identity data.
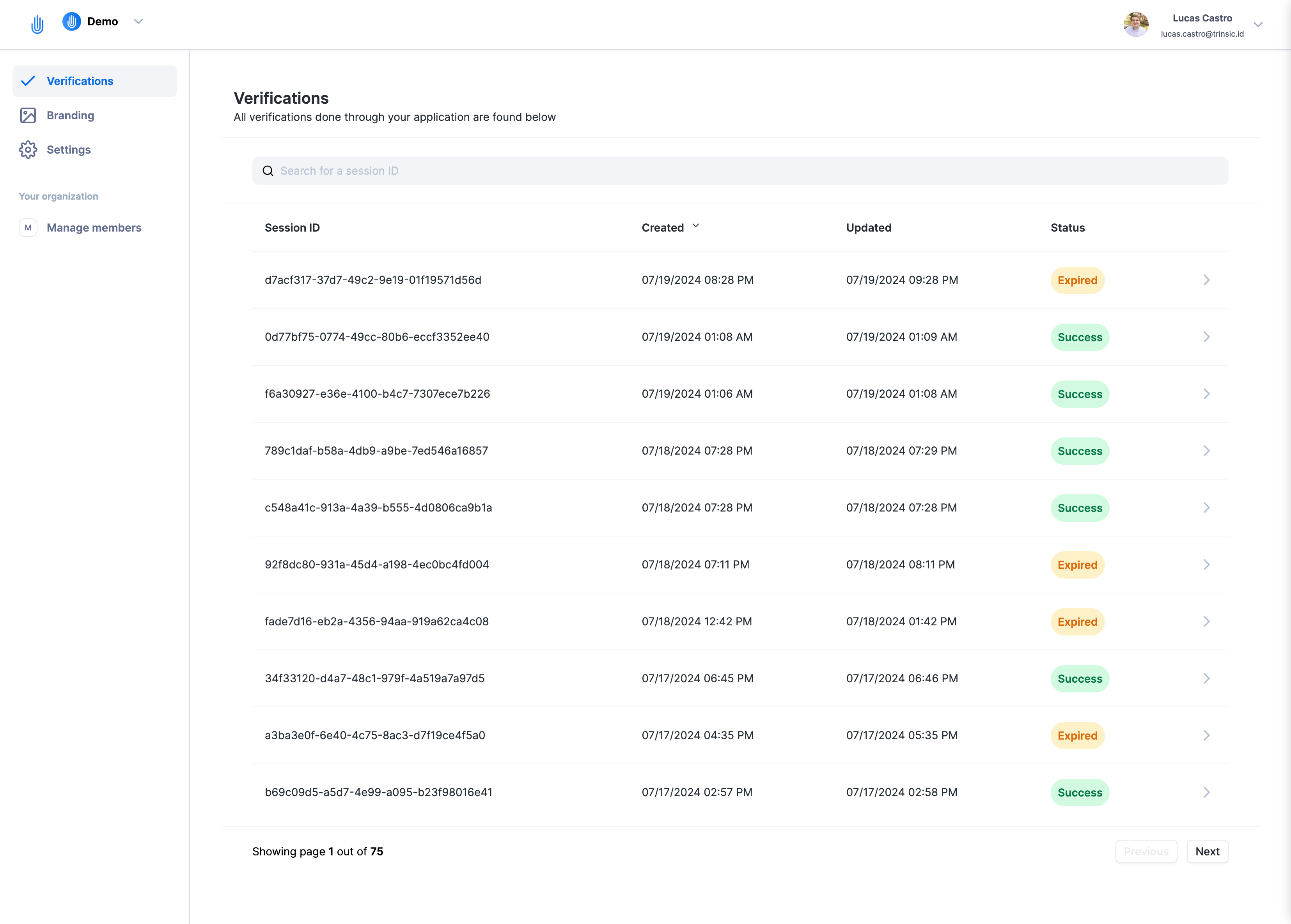
Conclusion
Congratulations on completing the guide to getting started with Trinsic! You have now established the groundwork for a seamless and user-friendly identity verification experience within your product.
Updated 2 months ago